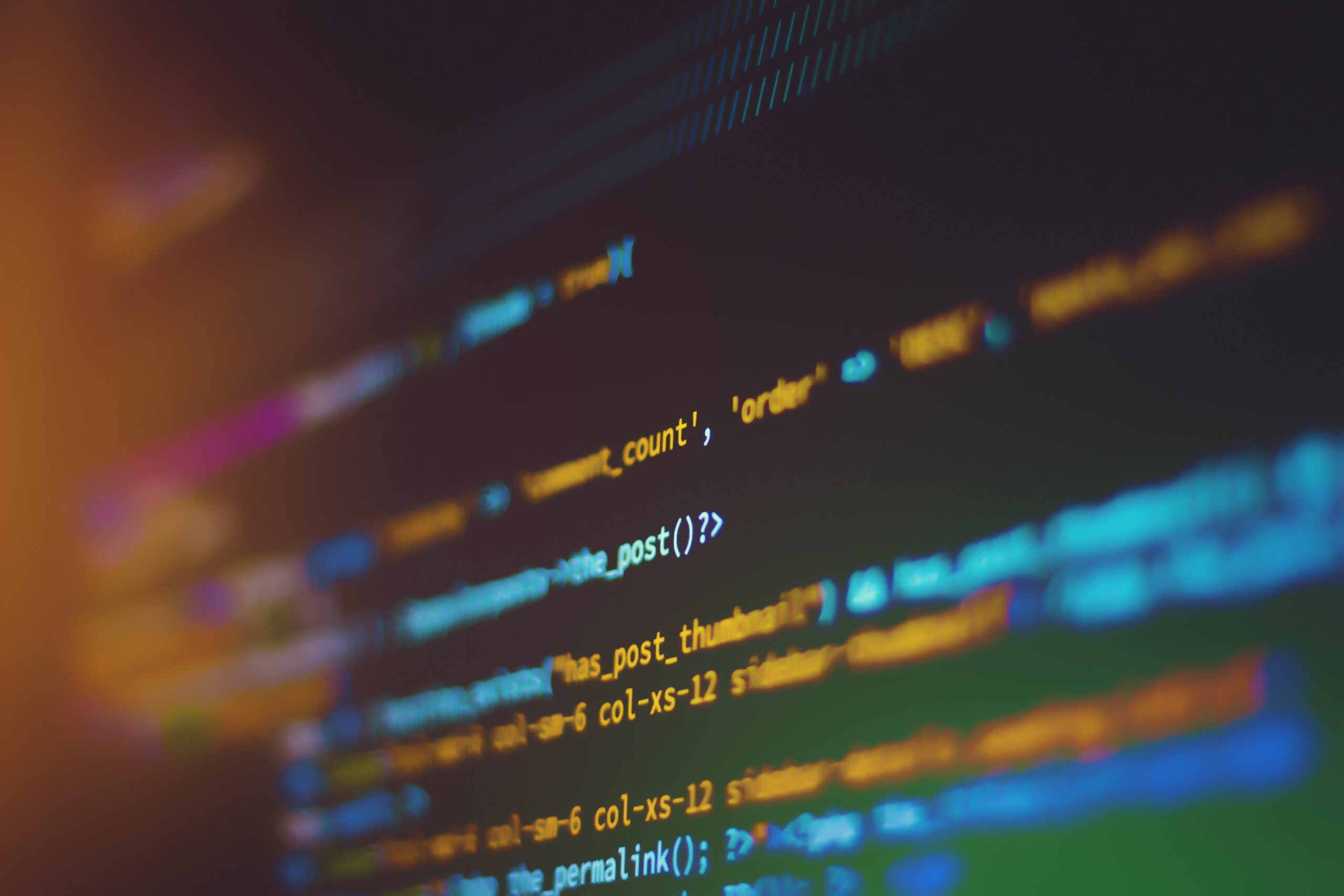
In today’s digital landscape, securing web applications has become more critical than ever. Developers must ensure that sensitive data is protected from unauthorized access and cyber threats. One of the key tools in achieving this is cryptography, which can be effectively implemented in web frameworks like Flask. This article explores how to integrate cryptography in Flask, the popular Python web framework, to build secure and resilient web applications.
Introduction to Flask and Cryptography
Flask is a lightweight and flexible web framework written in Python, known for its simplicity and ease of use. It’s a popular choice for developers looking to build small to medium-sized web applications quickly. However, as with any web application, security must be a priority, especially when handling sensitive data such as user credentials, financial information, or personal details.
Cryptography is the practice of securing information by transforming it into an unreadable format, known as encryption. Only those with the appropriate key can decrypt and access the information. In the context of web development, cryptography is essential for protecting data both at rest (stored data) and in transit (data being transmitted).
Why Use Cryptography in Flask?
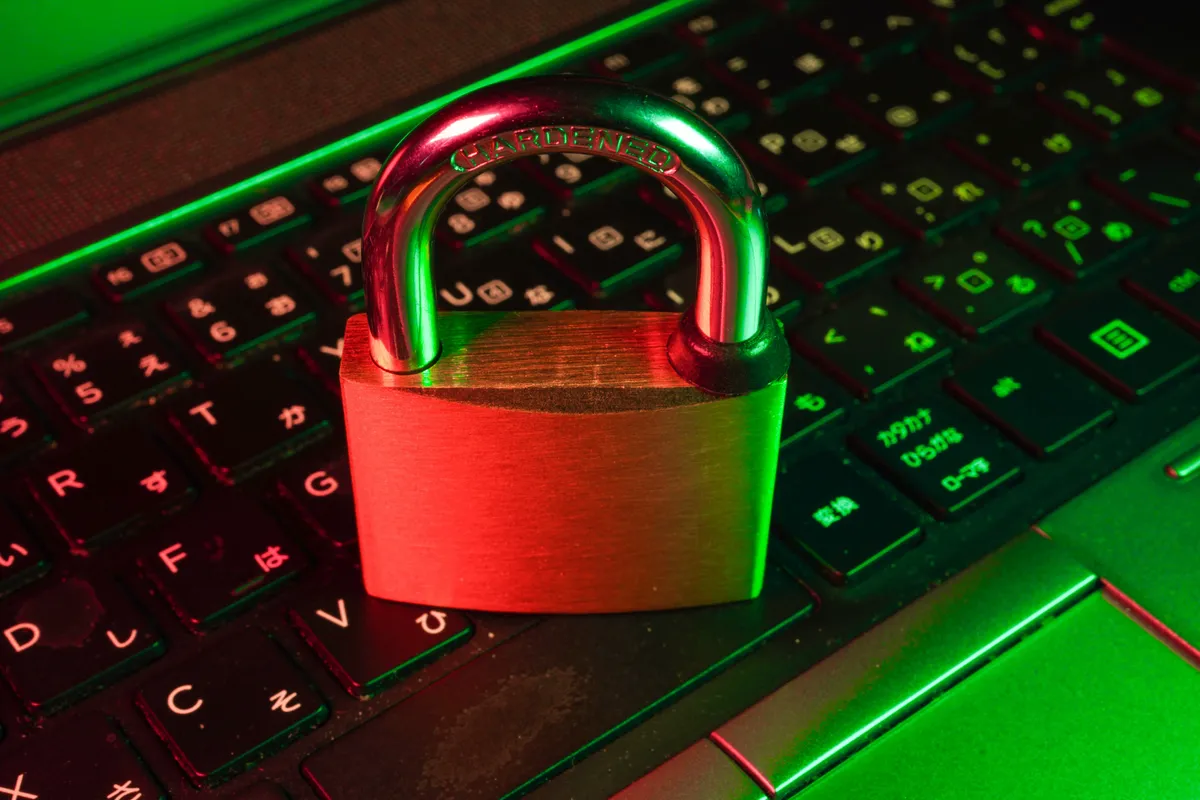
Cryptography in Flask can be used for various purposes, including:
- Data Encryption: Protecting sensitive data stored in databases or files.
- Password Hashing: Securing user passwords by hashing them before storing them in the database.
- Secure Communication: Ensuring that data transmitted between the client and server is encrypted to prevent eavesdropping or tampering.
- Token-Based Authentication: Creating secure tokens for session management or API authentication.
Setting Up Flask with Cryptography
To start using cryptography in Flask, you’ll need to install the necessary libraries. The two main Python libraries used for cryptography are cryptography
and Flask-Bcrypt
. Here’s how to get started:
pip install Flask cryptography Flask-Bcrypt
Once the libraries are installed, you can begin integrating cryptographic features into your Flask application.
Implementing Cryptography in Flask
1. Password Hashing with Flask-Bcrypt
Password hashing is a crucial aspect of securing user credentials. Instead of storing passwords in plaintext, Flask-Bcrypt allows you to hash passwords before saving them in the database. Here’s an example:
from flask import Flask, request, jsonify
from flask_bcrypt import Bcrypt
app = Flask(__name__)
bcrypt = Bcrypt(app)
@app.route('/register', methods=['POST'])
def register():
password = request.json['password']
hashed_password = bcrypt.generate_password_hash(password).decode('utf-8')
# Save hashed_password to the database
return jsonify({"message": "User registered successfully"}), 201
In this example, the password provided by the user is hashed using bcrypt.generate_password_hash()
before being stored in the database. The .decode('utf-8')
ensures that the hashed password is stored as a string.
2. Encrypting Data with Fernet
The cryptography
library provides a symmetric encryption tool called Fernet, which ensures that data can be securely encrypted and decrypted. Here’s how you can use Fernet in Flask:
from cryptography.fernet import Fernet
# Generate a key for encryption and decryption
key = Fernet.generate_key()
cipher_suite = Fernet(key)
# Encrypt data
def encrypt_data(data):
return cipher_suite.encrypt(data.encode('utf-8'))
# Decrypt data
def decrypt_data(encrypted_data):
return cipher_suite.decrypt(encrypted_data).decode('utf-8')
You can use the encrypt_data
and decrypt_data
functions to securely handle sensitive information within your Flask application.
3. Secure Communication with HTTPS
To ensure that data transmitted between the client and server is secure, it’s crucial to serve your Flask application over HTTPS. This can be achieved by configuring your web server (e.g., Nginx, Apache) with an SSL certificate.
Additionally, Flask can be configured to enforce HTTPS by redirecting HTTP requests to HTTPS:
from flask import Flask, redirect, request
app = Flask(__name__)
@app.before_request
def before_request():
if not request.is_secure:
return redirect(request.url.replace("http://", "https://"))
This simple middleware ensures that all requests are securely transmitted over HTTPS, protecting against man-in-the-middle attacks.
4. Token-Based Authentication
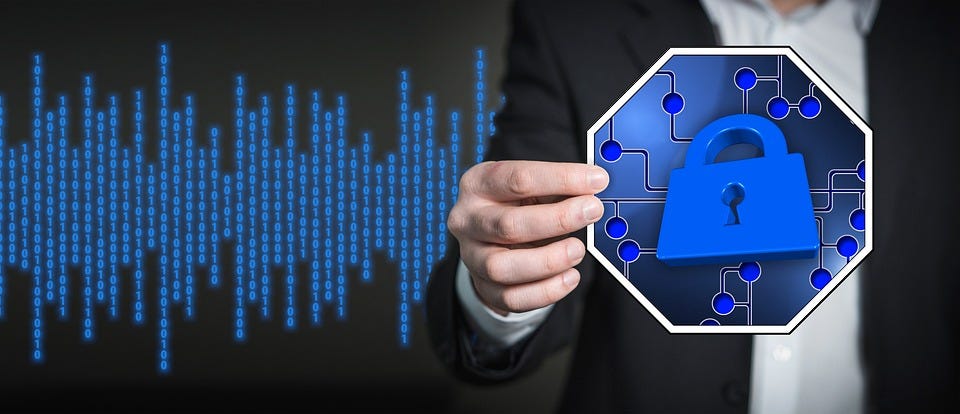
For APIs or session management, token-based authentication is a secure way to manage user sessions. You can use JSON Web Tokens (JWT) along with cryptographic techniques to generate and verify tokens:
import jwt
from datetime import datetime, timedelta
SECRET_KEY = 'your_secret_key'
def generate_token(user_id):
payload = {
'exp': datetime.utcnow() + timedelta(days=1),
'iat': datetime.utcnow(),
'sub': user_id
}
return jwt.encode(payload, SECRET_KEY, algorithm='HS256')
def decode_token(token):
try:
payload = jwt.decode(token, SECRET_KEY, algorithms=['HS256'])
return payload['sub']
except jwt.ExpiredSignatureError:
return None
except jwt.InvalidTokenError:
return None
With these functions, you can generate a JWT for authenticated users and verify it when they make subsequent requests.
Best Practices for Secure Flask Applications
- Keep Your Secrets Safe: Store your secret keys, encryption keys, and other sensitive information in environment variables or a secure vault, rather than hardcoding them in your application.
- Regularly Update Dependencies: Ensure that your cryptography libraries and Flask framework are regularly updated to protect against vulnerabilities.
- Use Strong Password Policies: Implement strong password policies in your application to encourage users to create secure passwords.
- Monitor and Audit: Regularly monitor and audit your application for any suspicious activities or security breaches.
Integrating cryptography into your Flask application is essential for building secure and resilient web applications. By leveraging tools like Flask-Bcrypt, Fernet, HTTPS, and JWTs, you can protect your users’ data and ensure secure communication between the client and server. As threats to web applications continue to evolve, staying informed about best practices in cryptography and web security will help you keep your Flask application secure.